Documentation
/API
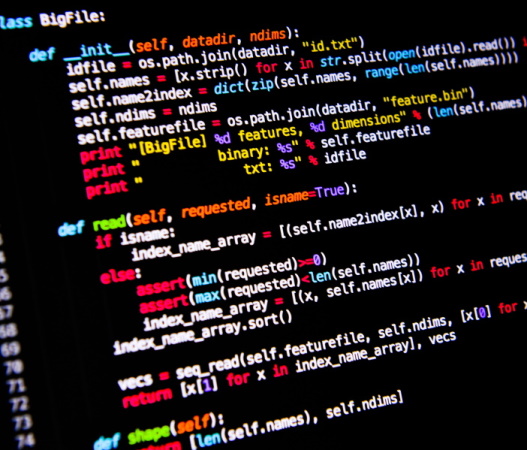
Interfacing with RF Power Nanny
You can communicate with RF Power Nanny and integrate it in your own code for custom functionality or your own UI or lab testing purposes. There are two main technology routes. If you have an Espressif device like an ESP32 variant, you can use ESP-NOW to interface with RF Power Nanny. You can think of ESP32 display devices. The other route is to use a HTTP connection with common web technology using Python, JS or any language capable of HTTP communication.

HTTP API
Communication using HTTP is done using HTTP Post requests for sending and SSE (Server-Side Events) for receiving information back. Only one POST endpoint (/power) that will return information directly. The other two endpoints (/set and /request) will not return info back. These are the endpoints:
- /power
- /set
- /request
Power endpoint
The /power endpoint is the only endpoint that will return information directly in the response. It can be used from the browser to get the current power reading back as a JSON object. Or you can use it in your code to fetch a reading. We will publish some Python code on GitHub as a reference.
The response has the following format:
{
"FWDMV" : float, // Forward power in mV
"REFMV" : float, // Reflected power in mV
"FREQ" : int, // Frequency in kHz
"MNR" : int, // Measurement number
"MS" : unsigned long, // Measurement time
"BTEMP" : float, // Bridge temperature
"CTEMP" : float // Controller temperature
}
see Power Formulas to convert mV to Watt or dBm
Set endpoint
The /set endpoint can be used to configure RF Power Nanny, frontend or “Look Who’s Talking”. The response object does not contain information. Values are posted using http query parameters (key value pairs) like /request?key=value. Our advice is to post only one parameter (key value pair) per request. The following settings can be done using the /set endpoint:
peak_input
Value: True (1) or False (0)
True: use Peak power
False: use Average power
sampletime_input
Value: integer
Sample time in ms for probing max power value
scale_input
Value: integer (only affects frontend)
Scale 10, 25, 50 or 100
Scale 0 means auto
vcal_input
Value: float (affects frontend and external ESP32 devices)
Frequency independant calibration adjustment
fcal_input
Value: float (affects frontend and external ESP32 devices)
Frequency dependant calibration adjustment
call_input
Value: string (only affects Look Who's Talking)
can not contain ',' - replace comma's with '_.~'
Callsign
latlong_input
Value: string (only affects Look Who's Talking)
format lat_.~long
can not contain ',' - replace comma's with '_.~'
lat/long coordinates of the shack (location of Power Nanny)
mess_input
Value: string (only affects Look Who's Talking)
can not contain ',' - replace comma's with '_.~'
short message (max length 50 characters) for activity
Request endpoint
The /request endpoint can be used to trigger an action or to trigger a SSE response. The response object does not contain information. Most values are empty (the “key” is posted with an empty value). Use http query parameters (key value pairs) like /request?key=value. Our advice is to post only one parameter (key value pair) per request. The following settings can be done using the /set endpoint:
restart
Value: empty
Restart Power Nanny
getVersion
Value: empty
SSE response: version
Get firmware version
update
Value: string (filename without extension)
Update firmware with given filename
resetADC
Value: empty
Reset the on-board ADC
getExtDevAddress
Value: empty
SSE response: mac address
Get mac address of paired ESP-NOW device
unpair
Value: empty
Unpair paired ESP-NOW device
listenPairing
Value: empty
Start listening for pairing request from ESP-NOW device
endListenPairing
Value: empty
Stop listening for pairing request from ESP-NOW device
acceptPeer
Value: empty
Accept ESP-NOW peer
getSettings
Value: empty
SSE response: settings comma delimited
Format: name_extension, vCal_input, fCal_input, peak_input, sampletime_input, internet_access, scale_input, call_input, latlong_input, mess_input
Get current settings
Server Side Events (SSE)
RF Power Nanny will publish Server Side Events you can listen for. Below are the events you can listen for:
- measurement
- temperature
- settings
- ext_dev_addr
- pairingRequest
- version
measurement
Data: power
Format: Forward power mV, Reflected power mV, Frequency kHz
temperature
Data: temperature
Format: Controller temperature °C, Bridge temperature °C
settings
Data: current settings
Format: name_extension, vCal_input, fCal_input, peak_input, sampletime_input, internet_access, scale_input, call_input, latlong_input, mess_input
ext_dev_addr
Data: mac address of external ESP-NOW device
pairingRequest
Data: peer identifier string
version
Data: version string
source = new EventSource(nannyUrl + '/events')
source.addEventListener('open', function(e) {
console.log("SSE - sse open event")
}, false)
source.addEventListener('error', function(e) {
console.log("SSE - sse error event")
}, false)
source.addEventListener('temperature', function(e) {
console.log("SSE - sse temperature event")
}, false)
source.addEventListener('measurement', function(e) {
console.log("SSE - sse measurement event")
}, false);
source.addEventListener('pairingRequest', function(e) {
console.log("SSE - sse pairingRequest event")
}, false);
source.addEventListener('ext_dev_addr', function(e) {
console.log("SSE - sse ext_dev_addr event")
}, false);
source.addEventListener('version', function(e) {
console.log("SSE - sse version event")
}, false);
source.addEventListener('settings', function(e) {
console.log("SSE - sse settings event")
}, false);
//
Power Formulas
Measurement data is communicated in mV only. The receiving device or system should translate this in the form most suitable. Below you will find a JS implementation for converting received data to Watt and dBm. Here the “powerData” string is the comma delimited string received with a measurement event. Note that for a specific frequency range the value is adjusted with a fixed rCal value of 7.7. This is needed as an extra correction due to a cavity/resonance effect. It improves the accuracy for this frequency range.
let pArr = powerData.split(",")
freq = (parseInt(pArr[2])/1000).toFixed(3) // frequency in kHz parsed as integer to MHz and then formatted with 3 decimals
fwdmV = parseFloat(pArr[0])
refmV = parseFloat(pArr[1])
let freqCorrection = fCal * Math.log10(parseInt(pArr[2])/1000)
if ((freq >= 5) && (freq < 15)) {
fwdWatt = Math.pow(10, (fwdmV - vCal + 7.7 + freqCorrection)/250) // 10^((mV-vCal+rCal+(LOG(fMHz)*fCal))/250)
refWatt = Math.pow(10, (refmV - vCal + 7.7 + freqCorrection)/250)
} else {
fwdWatt = Math.pow(10, (fwdmV - vCal + freqCorrection)/250) // 10^((mV-vCal+(LOG(fMHz)*fCal))/250)
refWatt = Math.pow(10, (refmV - vCal + freqCorrection)/250)
}
fwddBm = 10 * Math.log10(fwdWatt) + 30
refdBm = 10 * Math.log10(refWatt) + 30
//
ESP-NOW
When you want to use an external (display) device other then a computer or phone you can use any ESP32 powered terminal. The way RF Power Nanny can communicate with such a device is by using the ESP-NOW protocol. You can study the firmware of an ATOMS3R implementation to see how this can be done.
We will publish the firmware source code for the Power Nanny Extrenal Device Client for M5Stack ATOMS3R on GitHub as a reference: source code